---------------------------------------------------------------------------
UnimplementedError Traceback (most recent call last)
Cell In[29], line 9
3 train_generator = train_dataset.shuffle(len(train_Q1),
4 seed=7,
5 reshuffle_each_iteration=True).batch(batch_size=batch_size)
6 val_generator = val_dataset.shuffle(len(val_Q1),
7 seed=7,
8 reshuffle_each_iteration=True).batch(batch_size=batch_size)
----> 9 model = train_model(Siamese, TripletLoss,text_vectorization,
10 train_generator,
11 val_generator,
12 train_steps=train_steps,)
Cell In[28], line 30, in train_model(Siamese, TripletLoss, text_vectorizer, train_dataset, val_dataset, d_feature, lr, train_steps)
26 model.compile(loss=TripletLoss,
27 optimizer = tf.keras.optimizers.Adam(learning_rate=lr)
28 )
29 # Train the model
---> 30 model.fit(train_dataset,
31 epochs = train_steps,
32 validation_data = val_dataset,
33 )
35 ### END CODE HERE ###
37 return model
File /usr/local/lib/python3.8/dist-packages/keras/src/utils/traceback_utils.py:70, in filter_traceback.<locals>.error_handler(*args, **kwargs)
67 filtered_tb = _process_traceback_frames(e.__traceback__)
68 # To get the full stack trace, call:
69 # `tf.debugging.disable_traceback_filtering()`
---> 70 raise e.with_traceback(filtered_tb) from None
71 finally:
72 del filtered_tb
File /usr/local/lib/python3.8/dist-packages/tensorflow/python/eager/execute.py:53, in quick_execute(op_name, num_outputs, inputs, attrs, ctx, name)
51 try:
52 ctx.ensure_initialized()
---> 53 tensors = pywrap_tfe.TFE_Py_Exec
error - > dtype = tf.string
# GRADED FUNCTION: Siamese
def Siamese(text_vectorizer, vocab_size=36224, d_feature=128):
"""Returns a Siamese model.
Args:
text_vectorizer (TextVectorization): TextVectorization instance, already adapted to your training data.
vocab_size (int, optional): Length of the vocabulary. Defaults to 56400.
d_model (int, optional): Depth of the model. Defaults to 128.
Returns:
tf.model.Model: A Siamese model.
"""
### START CODE HERE ###
branch = tf.keras.models.Sequential(name='sequential')
# Add the text_vectorizer layer. This is the text_vectorizer you instantiated and trained before
branch.add(text_vectorizer)
# Add the Embedding layer. Remember to call it 'embedding' using the parameter `name`
branch.add(tf.keras.layers.Embedding(vocab_size, d_feature, name='embedding'))
# Add the LSTM layer, recall from W2 that you want to the LSTM layer to return sequences, ot just one value.
# Remember to call it 'LSTM' using the parameter `name`
branch.add(tf.keras.layers.LSTM(d_feature, return_sequences=True, name='LSTM'))
# Add the GlobalAveragePooling1D layer. Remember to call it 'mean' using the parameter `name`
branch.add(tf.keras.layers.GlobalAveragePooling1D(name='mean'))
# Add the normalizing layer using the Lambda function. Remember to call it 'out' using the parameter `name`
branch.add(tf.keras.layers.Lambda(lambda x: tf.math.l2_normalize(x, axis=1), name='out'))
# Define both inputs. Remember to call then 'input_1' and 'input_2' using the `name` parameter.
# Be mindful of the data type and size
input1 = tf.keras.layers.Input(shape=(1, ), dtype=tf.string, name='input_1')
input2 = tf.keras.layers.Input(shape=(1, ), dtype=tf.string, name='input_2')
# Define the output of each branch of your Siamese network. Remember that both branches have the same coefficients,
# but they each receive different inputs.
branch1 = branch(input1)
branch2 = branch(input2)
# Define the Concatenate layer. You should concatenate columns, you can fix this using the `axis`parameter.
# This layer is applied over the outputs of each branch of the Siamese network
conc = tf.keras.layers.Concatenate(axis=1, name='conc_1_2')([branch1, branch2])
### END CODE HERE ###
return tf.keras.models.Model(inputs=[input1, input2], outputs=conc, name="SiameseModel")
# GRADED FUNCTION: train_model
def train_model(Siamese, TripletLoss, text_vectorizer, train_dataset, val_dataset, d_feature=128, lr=0.01, train_steps=5):
"""Training the Siamese Model
Args:
Siamese (function): Function that returns the Siamese model.
TripletLoss (function): Function that defines the TripletLoss loss function.
text_vectorizer: trained instance of `TextVecotrization`
train_dataset (tf.data.Dataset): Training dataset
val_dataset (tf.data.Dataset): Validation dataset
d_feature (int, optional) = size of the encoding. Defaults to 128.
lr (float, optional): learning rate for optimizer. Defaults to 0.01
train_steps (int): number of epochs
Returns:
tf.keras.Model
"""
## START CODE HERE ###
# Instantiate your Siamese model
model = Siamese(text_vectorizer,
vocab_size = text_vectorizer.vocabulary_size(), #set vocab_size accordingly to the size of your vocabulary
d_feature = d_feature)
# Compile the model
model.compile(loss=TripletLoss,
optimizer = tf.keras.optimizers.Adam(learning_rate=lr)
)
# Train the model
model.fit(train_dataset,
epochs = train_steps,
validation_data = val_dataset,
)
### END CODE HERE ###
return model
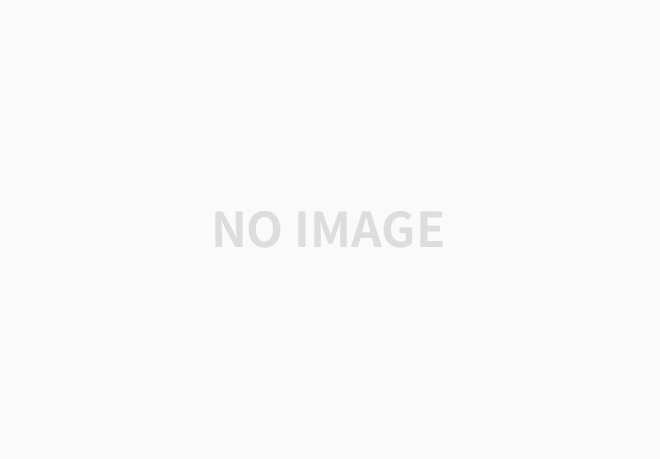
working